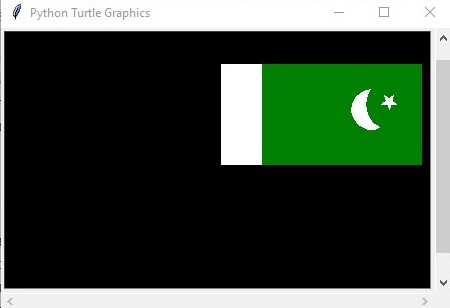 |
Python Turtle Graphics showing Pakistani Flag |
Python Code
import
turtle
#
Create a turtle object
flag
= turtle.Turtle()
#
Set the background color to white
turtle.bgcolor("black")
#
Draw the green rectangle
flag.begin_fill()
flag.color("green")
flag.forward(200)
flag.left(90)
flag.forward(100)
flag.left(90)
flag.forward(200)
flag.left(90)
flag.forward(100)
flag.end_fill()
#
Draw the white rectangle
flag.penup()
flag.goto(0,100)
flag.pendown()
flag.color("white")
flag.begin_fill()
flag.forward(100)
flag.left(90)
flag.forward(40)
flag.left(90)
flag.forward(100)
flag.left(90)
flag.forward(40)
flag.end_fill()
#
Draw the crescent
flag.penup()
flag.goto(150, 75)
flag.pendown()
flag.color("white")
flag.begin_fill()
flag.circle(20)
flag.end_fill()
flag.penup()
flag.goto(170, 85)
flag.pendown()
flag.color("green")
flag.begin_fill()
flag.circle(25)
flag.end_fill()
#
Draw the star
flag.penup()
flag.goto(175, 60)
flag.pendown()
flag.color("white")
flag.begin_fill()
for
i
in
range(5):
flag.forward(15)
flag.right(144)
flag.end_fill()
#
Hide the turtle
flag.hideturtle()
#
Keep the window open
turtle.done()
Explanation of the Code
This code uses the Python turtle
library to draw the flag of Pakistan. The turtle library allows you to create
graphics using a turtle object that you can move around the screen. Here is a
step-by-step explanation of the code:
1. The
first line imports the turtle library, which is necessary to use the turtle
object and its methods.
2.
Next,
a turtle object named "flag" is created.
3.
The
background color of the window is set to black by calling
turtle.bgcolor("black").
4.
The
flag object begins drawing the green rectangle by calling flag.begin_fill() and
setting the color to green using flag.color("green"). The flag object
then moves forward by 200 units, turns left by 90 degrees, moves forward by 100
units, turns left again by 90 degrees, moves forward by 200 units, and turns
left one last time by 90 degrees. The flag object then moves forward by 100
units and ends the fill with flag.end_fill().
5.
The
flag object then moves to the top left corner of the white rectangle by calling
flag.penup(), flag.goto(0,100), and flag.pendown(). It then sets the color to
white and begins filling the rectangle by calling flag.color("white")
and flag.begin_fill(). The flag object then moves forward by 100 units, turns
left by 90 degrees, moves forward by 40 units, turns left again by 90 degrees,
moves forward by 100 units, and turns left one last time by 90 degrees. The
flag object then moves forward by 40 units and ends the fill with
flag.end_fill().
6.
The
flag object then moves to the middle of the crescent by calling flag.penup(),
flag.goto(150, 75), and flag.pendown(). It then sets the color to white and
begins filling the crescent by calling flag.color("white") and
flag.begin_fill(). The flag object then draws a circle with a radius of 20
units using flag.circle(20) and ends the fill with flag.end_fill(). The flag
object then moves to the right of the crescent by calling flag.penup(),
flag.goto(170, 85), and flag.pendown(). It then sets the color to green and
begins filling the crescent by calling flag.color("green") and flag.begin_fill().
The flag object then draws a circle with a radius of 25 units using
flag.circle(25) and ends the fill with flag.end_fill().
7.
The
flag object then moves to the middle of the star by calling flag.penup(),
flag.goto(175, 60), and flag.pendown(). It then sets the color to white and
begins filling the star by calling flag.color("white") and
flag.begin_fill(). The flag object then uses a for loop to move forward 15
units and turn right by 144 degrees, five times. This creates the shape of the
star. The flag object then ends the fill with flag.end_fill().
8.
The
flag object is then hidden from the screen by calling flag.hideturtle().
9.
The
turtle window is kept open by calling turtle.done().
10.
This
code will draw Pakistan flag on turtle window. you can run this code on your
python IDE and you can see the output flag of Pakistan.
Comments
Post a Comment